Stored Procedures are a powerful tool in the world of database management, offering efficiency and convenience. Imagine having a set of instructions readily available that can be executed with just a simple call, eliminating the need to repeatedly write complex queries. In this article, we will explore the benefits of stored procedures and how they can enhance the functionality of your database systems. So sit back, relax, and let us delve into the world of stored procedures together.
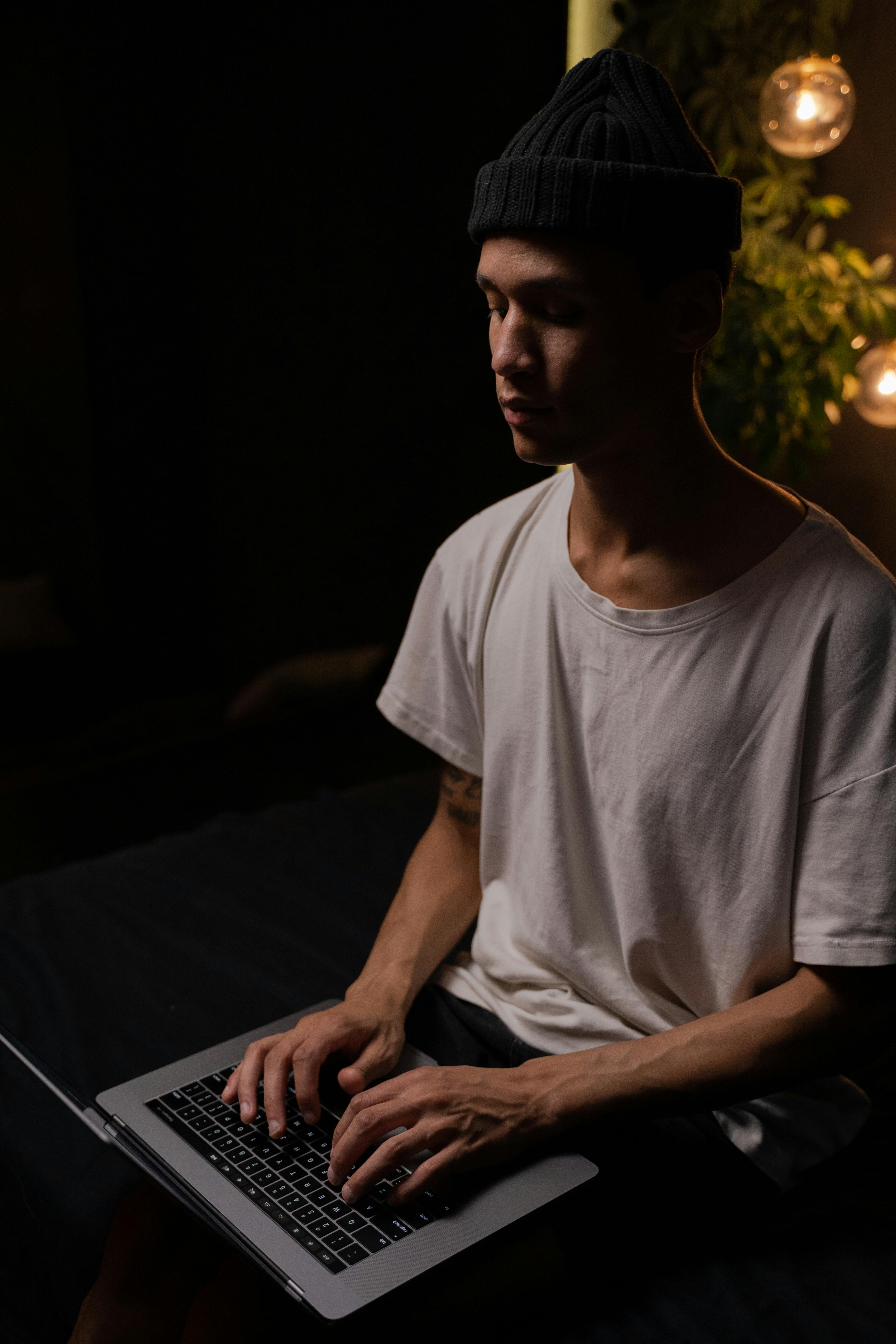
What are Stored Procedures?
Definition
Stored procedures are a collection of precompiled SQL statements and procedural logic that are stored in a database. They are designed to perform specific tasks and can be created, called, modified, and dropped as needed.
Purpose
The purpose of using stored procedures is to provide a convenient way to execute frequently used SQL queries and routines. They help improve performance, code reusability, security, maintenance, and network bandwidth optimization. By consolidating repeated SQL code into a stored procedure, developers can simplify their application code and enhance the overall efficiency of their database operations.
Advantages of Stored Procedures
Improved Performance
One of the key advantages of stored procedures is improved performance. Since the SQL code in a stored procedure is precompiled and stored in the database, it can be executed much faster than ad-hoc queries. This is because the execution plan is created once and reused multiple times, eliminating the need for repetitive compilation of SQL statements.
Code Reusability
Stored procedures promote code reusability by allowing developers to encapsulate frequently executed SQL code into a single procedure. This means that the same piece of code can be used by multiple applications or modules within an application, reducing the need for duplicating code and increasing overall efficiency and maintainability.
Security
Stored procedures can enhance security by providing controlled access to the underlying database. Instead of granting individual users direct access to tables and views, developers can create stored procedures that perform the necessary data operations. Users can then execute these procedures without needing direct access to the underlying objects, ensuring that sensitive data is protected and reducing the risk of unauthorized data modifications.
Maintenance
Stored procedures help ease the maintenance process by centralizing database logic. When changes or updates need to be made, developers only need to modify the relevant stored procedure rather than updating multiple instances of the same code scattered throughout the application. This saves time and effort, reduces the chance of introducing errors, and ensures consistency within the database.
Network Bandwidth Optimization
Another advantage of stored procedures is network bandwidth optimization. Instead of transmitting large amounts of data between the application and the database, stored procedures can be used to perform data processing on the server side. This reduces the volume of data that needs to be transmitted over the network, resulting in improved performance and reduced network traffic.https://www.youtube.com/embed/NrBJmtD0kEw
Creating Stored Procedures
Syntax
The syntax for creating a stored procedure varies depending on the database management system being used. However, a typical stored procedure declaration includes a name, parameters (if any), and the SQL code or procedural logic to be executed. For example, in SQL Server, the basic syntax for creating a stored procedure is as follows:
CREATE PROCEDURE procedure_name @parameter1 datatype1 = default_value1, @parameter2 datatype2 = default_value2 AS BEGIN — SQL code or procedural logic END
Parameters
Stored procedures can accept parameters, which are placeholders for input values passed to the procedure at runtime. Parameters allow developers to create flexible and reusable procedures that can perform different operations based on the input values provided. Parameters can be defined as input, output, or input/output, and can have default values assigned to them.
Variables
Variables are used in stored procedures to store and manipulate data during procedure execution. They provide a way to temporarily hold values and perform calculations within the procedure. Variables can be declared and assigned values within the procedure, and their scope is limited to the procedure in which they are defined.
Flow Control
Flow control statements, such as IF-ELSE and WHILE, can be used within stored procedures to control the execution path based on specific conditions. These statements allow developers to implement conditional logic and repetitive tasks within the procedure, enhancing its flexibility and functionality.
Error Handling
Error handling is an important aspect of stored procedures. It allows developers to gracefully handle errors and exceptions that may occur during procedure execution. By using try-catch blocks or error handling functions, developers can capture and handle errors appropriately, ensuring that the procedure behaves as expected and providing meaningful error messages to users.
Calling Stored Procedures
From SQL Statements
Stored procedures can be called from SQL statements using the EXEC or EXECUTE keyword, followed by the name of the procedure and any required parameters. For example, in SQL Server:
EXEC procedure_name @parameter1 = value1, @parameter2 = value2
From Application Code
Stored procedures can also be called from application code written in programming languages such as Java, C#, or Python. Application code can connect to the database, pass parameter values, and execute the stored procedure using the appropriate database APIs or libraries.
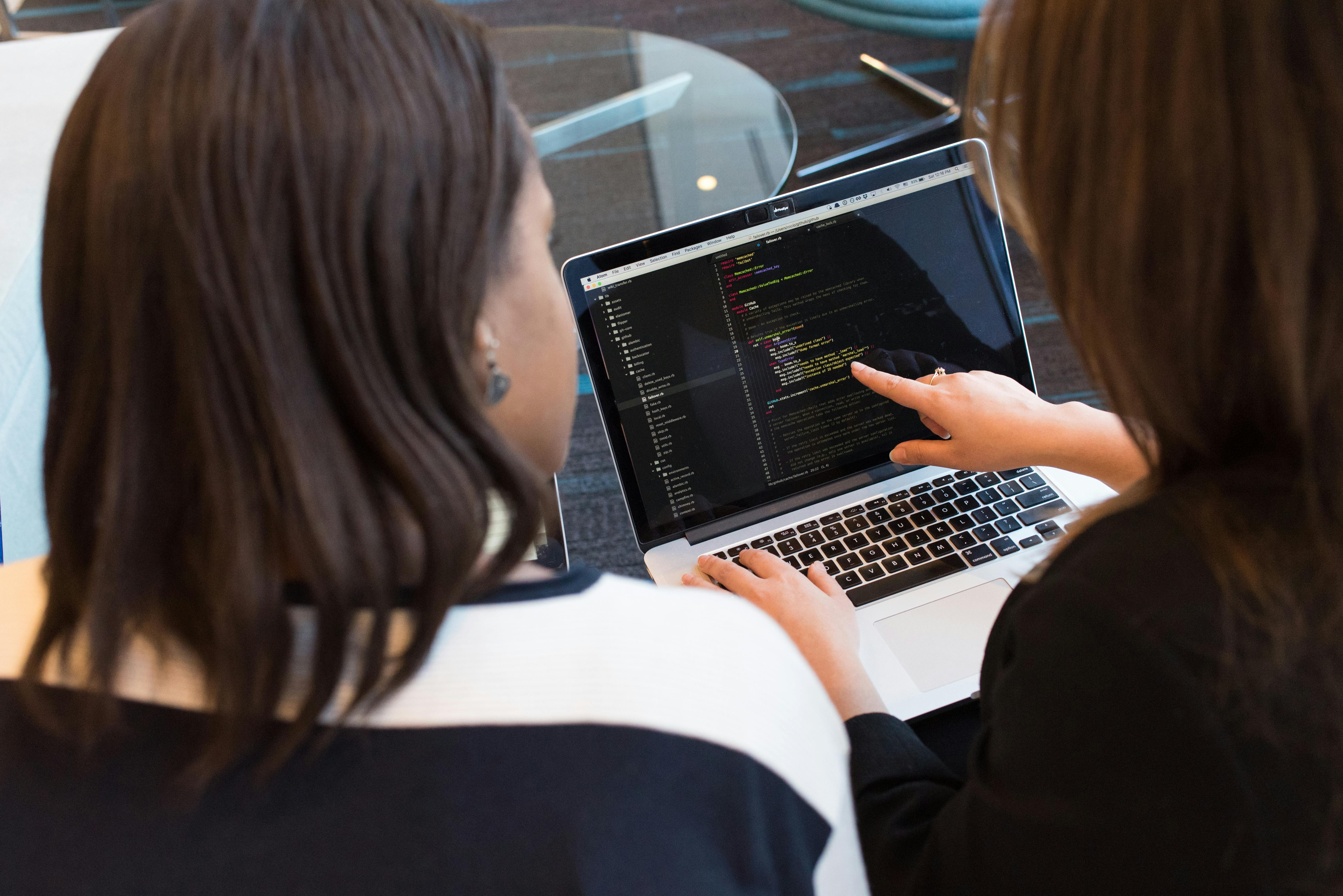
Modifying Stored Procedures
Altering Stored Procedures
Stored procedures can be modified using the ALTER PROCEDURE statement. This allows developers to make changes to the code or logic within an existing procedure without having to drop and recreate it. Modifying a stored procedure can involve updating the procedure’s SQL code, adding or removing parameters, or modifying the flow control and error handling logic.
Dropping Stored Procedures
If a stored procedure is no longer needed or if it needs to be recreated from scratch, it can be dropped using the DROP PROCEDURE statement. Dropping a stored procedure removes it from the database, ensuring that it can no longer be called or executed.
Using Stored Procedures in Different Database Management Systems
Stored procedures can be used in various database management systems, although the specific syntax and features may vary. Here are some examples of how stored procedures are used in different systems:
MySQL
In MySQL, stored procedures can be created using the CREATE PROCEDURE statement. Parameters can be defined using the IN, OUT, or INOUT keywords to specify their behavior. MySQL also supports flow control statements such as IF-ELSEIF-ELSE and CASE, as well as error handling using the SIGNAL statement.
Microsoft SQL Server
In SQL Server, stored procedures can be created using the CREATE PROCEDURE statement, similar to MySQL. SQL Server supports input, output, and input/output parameters, as well as the ability to return result sets. It also offers advanced features such as transaction management and table-valued parameters.
Oracle
In Oracle, stored procedures are created using the CREATE PROCEDURE statement. Oracle allows the use of input, output, and input/output parameters, as well as the ability to define custom data types. Oracle also provides advanced features like exception handling using the EXCEPTION keyword and the ability to define autonomous transactions.
PostgreSQL
In PostgreSQL, stored procedures can be created using the CREATE PROCEDURE or CREATE FUNCTION statements. PostgreSQL supports input and output parameters, as well as the ability to return result sets. It also offers advanced features such as support for multiple languages (including PL/pgSQL and PL/Python) and the ability to define custom aggregate functions.
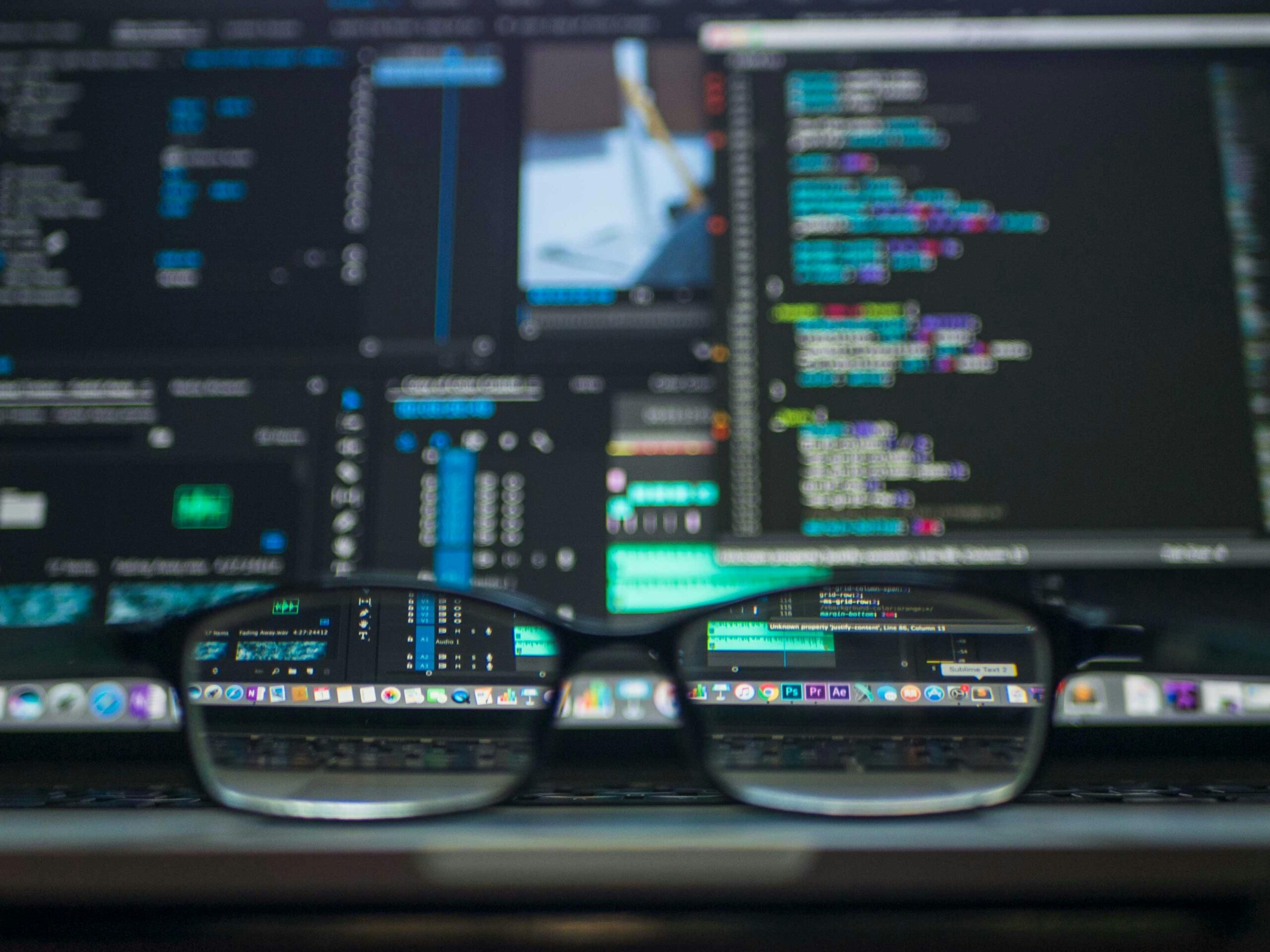
Stored Procedures Best Practices
Minimize Processing
To ensure optimal performance, it is important to minimize the processing within stored procedures. This means avoiding unnecessary calculations, iterations, or data manipulations that could slow down the procedure execution. Keeping the code concise and focused on the task at hand can help improve performance and efficiency.
Avoid Lengthy Procedures
Long and complex procedures can be more difficult to understand, maintain, and troubleshoot. It is best to keep stored procedures as short and simple as possible, focusing on a single task or operation. If a procedure starts to become too lengthy or complicated, consider breaking it down into smaller procedures or functions for better organization and readability.
Avoid Excessive Parameters
Using too many parameters in a stored procedure can make it harder to understand and use. It is important to only include necessary parameters and avoid excessive complexity. If a procedure requires a large number of inputs or outputs, consider using other techniques such as returning result sets or using temporary tables to reduce the number of parameters.
Document and Comment
Documenting stored procedures is crucial for understanding their purpose, behavior, and usage. It is important to provide clear and comprehensive documentation, including information on the inputs, outputs, and expected behavior of the procedure. Additionally, adding comments within the code can help other developers understand the logic and make future modifications easier.
Use Proper Naming Convention
Using a consistent and meaningful naming convention for stored procedures is important for clarity and organization. Choose descriptive names that accurately represent the purpose or action of the procedure. This will make it easier for developers to understand and locate the procedures when working with the database.
Real-life Use Cases of Stored Procedures
Data Validation and Manipulation
Stored procedures are commonly used for data validation and manipulation tasks. For example, a stored procedure can be created to validate and sanitize user input before inserting data into a database. It can also perform complex data transformations or calculations, such as generating summary reports or aggregating data from multiple tables.
Reporting and Analytics
Stored procedures can be used to generate reports and perform analytics on large amounts of data. By encapsulating complex SQL queries and calculations into a stored procedure, developers can simplify the reporting process and improve performance. Stored procedures can be scheduled to run regularly, generating updated reports or analytics results automatically.
Scheduling and Automation
Stored procedures can be used for scheduling and automating database tasks. For example, a stored procedure can be created to run at a specific time to perform data backups or apply database maintenance tasks. By scheduling these procedures to run automatically, developers can save time and ensure that critical tasks are performed regularly without manual intervention.
Common Mistakes with Stored Procedures
Nested Cursors
Using nested cursors within a stored procedure can lead to poor performance and inefficient code. Cursors are a powerful feature for processing rows one by one, but using them unnecessarily or inappropriately can result in excessive round trips to the database and increased overhead. It is best to avoid nested cursors when possible and look for alternative approaches such as set-based operations.
Lack of Error Handling
Failing to implement proper error handling in stored procedures can lead to unexpected behavior and difficult debugging. It is important to include adequate error handling logic within the procedure to capture and handle any potential errors or exceptions. This ensures that the procedure behaves as expected and provides meaningful error messages to users or application code.
Poorly Designed Parameters
Ineffective use of parameters can make stored procedures harder to understand and use. It is important to carefully design and organize the parameters to ensure clarity and simplicity. Using descriptive and meaningful parameter names, assigning appropriate data types, and avoiding excessive or unnecessary parameters can greatly enhance the usability and maintainability of the procedure.
Overuse or Misuse
Using stored procedures excessively or inappropriately can lead to unnecessary complexity and maintenance overhead. While stored procedures offer many benefits, it is important to use them judiciously and consider alternatives when appropriate. Not every SQL query or routine needs to be encapsulated in a stored procedure, and overusing them can lead to a bloated and complicated database architecture.
Conclusion
Stored procedures provide a powerful mechanism for managing and executing SQL code in a database. They offer numerous advantages such as improved performance, code reusability, security, maintenance, and network bandwidth optimization. By creating, calling, modifying, and using stored procedures effectively, developers can enhance the functionality, performance, and security of their databases. Following best practices and avoiding common mistakes can ensure that stored procedures are a valuable tool in the development and management of database systems.
Leave a Reply