magine a world where your database can gain superpowers – where complex tasks are executed seamlessly, and your data management becomes a breeze. This is where stored procedures come into play. Stored procedures are like your personal assistants, carrying out predefined sets of instructions with remarkable efficiency. Whether it’s extracting data, performing calculations, or handling multiple transactions, these powerful procedures are designed to make your life easier. Let’s explore how stored procedures can revolutionize your database management, empowering you to achieve more with less effort.https://www.youtube.com/embed/NrBJmtD0kEw
What are Stored Procedures?
Definition
Stored procedures are sets of pre-compiled SQL statements stored in a database. They are stored as a single unit, making it easier to manage and execute complex database operations. Essentially, a stored procedure is like a subroutine or a function in programming, but in the context of a database.
Purpose
The purpose of stored procedures is to provide a way to encapsulate frequently used database operations. By creating reusable and modular procedures, you can simplify complex queries and operations, improve performance, and enhance security. Stored procedures also promote code reusability and maintainability by separating business logic from application code.
Advantages
There are several advantages to using stored procedures:
- Improved Performance: Stored procedures are pre-compiled and optimized, resulting in faster execution times compared to executing individual SQL statements. The stored procedure’s execution plan is cached, reducing the overhead of query compilation.
- Security: Stored procedures help mitigate security risks by allowing fine-grained access control. Users can be granted permissions to execute stored procedures without granting direct access to underlying tables or database objects.
- Code Reusability: By encapsulating complex logic into stored procedures, you can reuse them across multiple applications or modules within an application. This saves development time and effort, and ensures consistency in data manipulation and calculations.
- Modularity and Maintenance: Stored procedures promote modular code design, making it easier to maintain and update database logic. Changes to the underlying database structure can be made within the stored procedure code, reducing the need for modifying application code.
- Transaction Management: Stored procedures can be used to manage transactions, ensuring atomicity, consistency, isolation, and durability (ACID properties) of database operations. This simplifies complex transactional workflows and reduces the chances of data inconsistencies.
- Data Integrity: Stored procedures allow you to enforce business rules and data validation constraints at the database level. This helps maintain data integrity by preventing invalid or inconsistent data from being inserted or modified.
Creating and Using Stored Procedures
Syntax
The syntax for creating a stored procedure varies depending on the database management system (DBMS) you are using. Typically, a stored procedure begins with the CREATE PROCEDURE
statement, followed by the procedure name, input parameters (if any), and the SQL code that defines the procedure’s logic.
For example, in Microsoft SQL Server:
CREATE PROCEDURE GetEmployeeDetails @EmployeeID INT AS BEGIN — SQL code to fetch employee details using the provided EmployeeID END
Parameterized Stored Procedures
Stored procedures can accept input parameters, which allow you to pass values to the procedure at runtime. Parameters provide flexibility and allow for customization of the procedure’s behavior. They can be of different data types, such as integers, strings, dates, or even custom-defined types.
For example, the previously mentioned stored procedure can be modified to accept an @EmployeeID
parameter:
CREATE PROCEDURE GetEmployeeDetails @EmployeeID INT AS BEGIN — SQL code to fetch employee details using the provided EmployeeID SELECT * FROM Employees WHERE EmployeeID = @EmployeeID; END
Executing Stored Procedures
Once a stored procedure is created, it can be executed using the EXECUTE
or EXEC
statement, followed by the procedure name and any required input parameters.
For example:
EXECUTE GetEmployeeDetails @EmployeeID = 123;
Alternatively, you can also use the shorter form:
EXEC GetEmployeeDetails 123;
Executing a stored procedure triggers the code defined within the procedure, which could involve database queries, updates, or any other supported SQL operations.
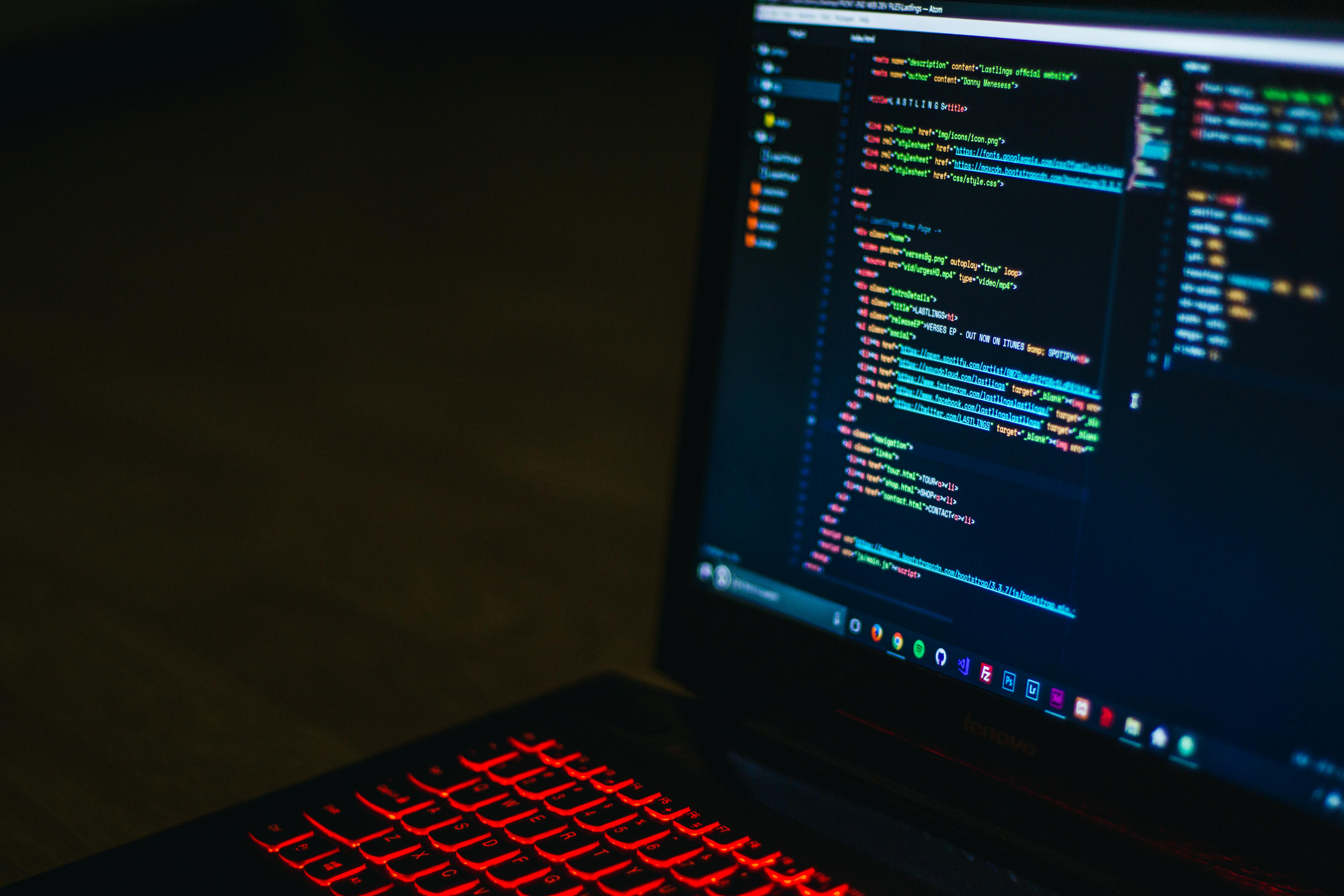
Managing Stored Procedures
Altering Stored Procedures
After creating a stored procedure, you may occasionally need to modify its behavior or add new functionality. In such cases, you can alter the stored procedure using the ALTER PROCEDURE
statement. This allows you to modify the procedure’s code, add or remove parameters, or make other necessary changes.
ALTER PROCEDURE GetEmployeeDetails @EmployeeID INT, @IncludeAddress BIT = 0 AS BEGIN — SQL code to fetch employee details using the provided EmployeeID SELECT EmployeeID, FirstName, LastName, Address FROM Employees WHERE EmployeeID = @EmployeeID;
IF @IncludeAddress = 1 BEGIN — Additional code to fetch employee address END END
Dropping Stored Procedures
If a stored procedure is no longer needed or if you wish to recreate it, you can drop it using the DROP PROCEDURE
statement. This removes the stored procedure from the database.
DROP PROCEDURE GetEmployeeDetails;
It is important to be cautious when dropping stored procedures, as it can impact dependent applications or scripts that rely on their existence.
Version Control for Stored Procedures
To manage the development and evolution of stored procedures, it is recommended to use version control systems such as Git or Subversion. By treating stored procedures as source code files, you can track changes, collaborate with other developers, and roll back to previous versions if needed. Version control also helps in maintaining an audit trail and facilitates code review and release management processes.
Security Concerns
Access Control
One important aspect of using stored procedures is controlling access to them. Access control ensures that only authorized users or applications can execute specific stored procedures and safeguard the confidentiality and integrity of the data.
Database systems offer various mechanisms for access control, such as using roles, users, and granting privileges at a granular level. It is crucial to grant necessary permissions to users or roles and always follow the principle of least privilege, providing only the required access to stored procedures and underlying data.
Injection Attacks
Stored procedures can help mitigate the risk of SQL injection attacks, a common security vulnerability where an attacker manipulates input parameters to execute unintended SQL code. By using parameterized stored procedures, where user input is treated as parameters rather than concatenated directly into SQL statements, you can prevent malicious SQL injection attacks.
Additionally, database systems often provide built-in features like bind variables or prepared statements, which further enhance security by automatically sanitizing and validating user input.
Data Leakage and Confidentiality
Stored procedures play a crucial role in maintaining data confidentiality. By encapsulating sensitive logic within stored procedures and restricting direct table access, unauthorized users or applications are prevented from querying or modifying sensitive data.
Furthermore, stored procedures allow for the implementation of data access policies, such as row-level security or column-level encryption, to safeguard data confidentiality. These policies ensure that only authorized users can access specific subsets of data, limiting the risk of data leakage.
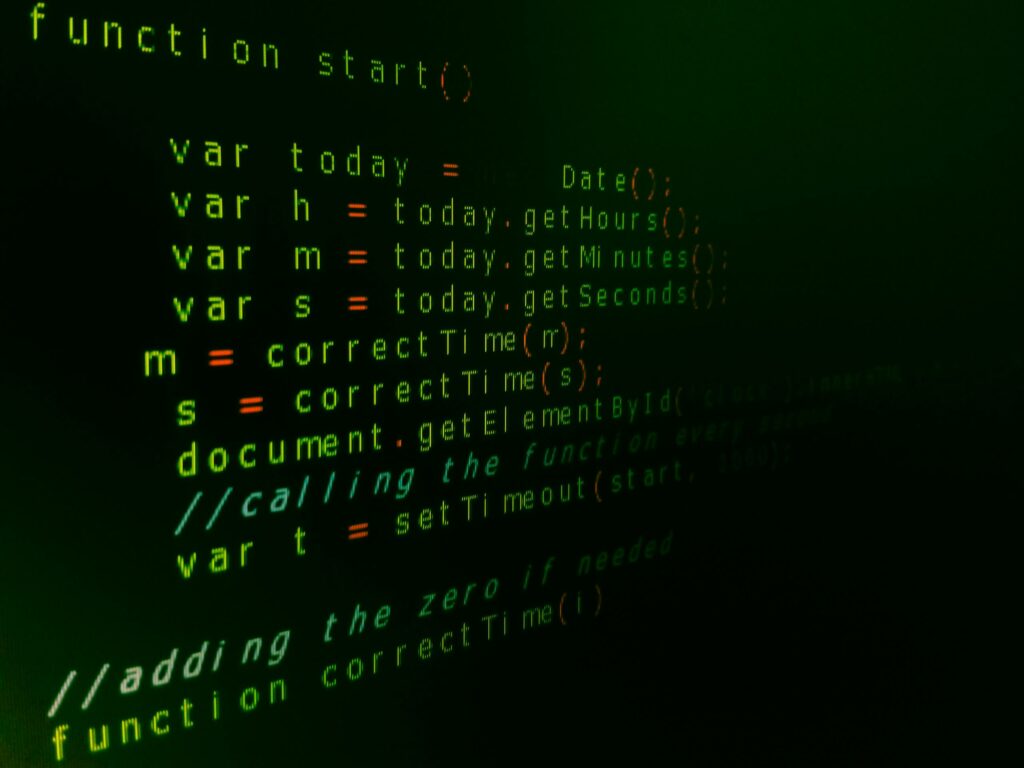
Best Practices for using Stored Procedures
Modularity and Reusability
To maximize the benefits of stored procedures, it is essential to adopt modularity and reusability principles. Design stored procedures to encapsulate specific functionality or business logic, making them reusable across multiple applications or modules.
By breaking down complex operations into smaller, well-defined procedures, you enhance code readability, maintainability, and the ability to easily modify or extend functionality without impacting the entire system. Aim to minimize code duplication and promote code reuse to improve development efficiency and consistency.
Error Handling
Proper error handling is critical in stored procedures to ensure robust and resilient database operations. Define clear error handling routines within your stored procedures to gracefully handle exceptions, handle transaction rollbacks when necessary, and provide meaningful error messages or log entries.
Consider using structured exception handling mechanisms provided by the database system, such as TRY-CATCH blocks, to capture and handle errors effectively. This helps in producing concise error logs, troubleshooting issues efficiently, and ensuring data integrity within transactional workflows.
Documentation
Documenting stored procedures is crucial for ensuring maintainability and promoting collaboration among developers. Document the purpose, input parameters, return values, and any relevant constraints or assumptions associated with each stored procedure.
By maintaining up-to-date documentation, developers can better understand the purpose and behavior of stored procedures, reducing the time required for troubleshooting and development. Good documentation also facilitates knowledge sharing and promotes best practices within the development team.
Performance Considerations
Query Execution Plan
Understanding and optimizing query execution plans is essential for ensuring optimal performance of stored procedures. The query execution plan describes the order and method in which the database engine executes the steps involved in executing a query.
Use tools provided by the database management system to analyze query execution plans, identify performance bottlenecks, and optimize query performance. Techniques such as creating appropriate indexes, rewriting queries, or utilizing database-specific features like materialized views can significantly enhance stored procedure performance.
Caching
Caching is an effective technique to improve the performance of stored procedures. Many database systems have built-in mechanisms to cache query results or compiled stored procedures, reducing the need for repetitive compilation or disk I/O.
Consider implementing caching strategies, such as using in-memory caches or leveraging caching frameworks like Redis, to store frequently accessed data or query results. Caching can significantly reduce the load on the database server and improve the response times of applications that rely on stored procedures.
Indexing
Proper indexing is crucial for optimizing the performance of stored procedures. Indexes facilitate fast data retrieval by creating ordered data structures based on specific columns. They speed up query execution by allowing the database engine to efficiently narrow down the search space.
Carefully analyze the queries used in stored procedures and identify the frequently accessed columns or join conditions. Based on this analysis, design and create appropriate indexes to improve query performance. However, be mindful of the trade-off between write performance and read performance, as indexes introduce overhead during data modification operations.
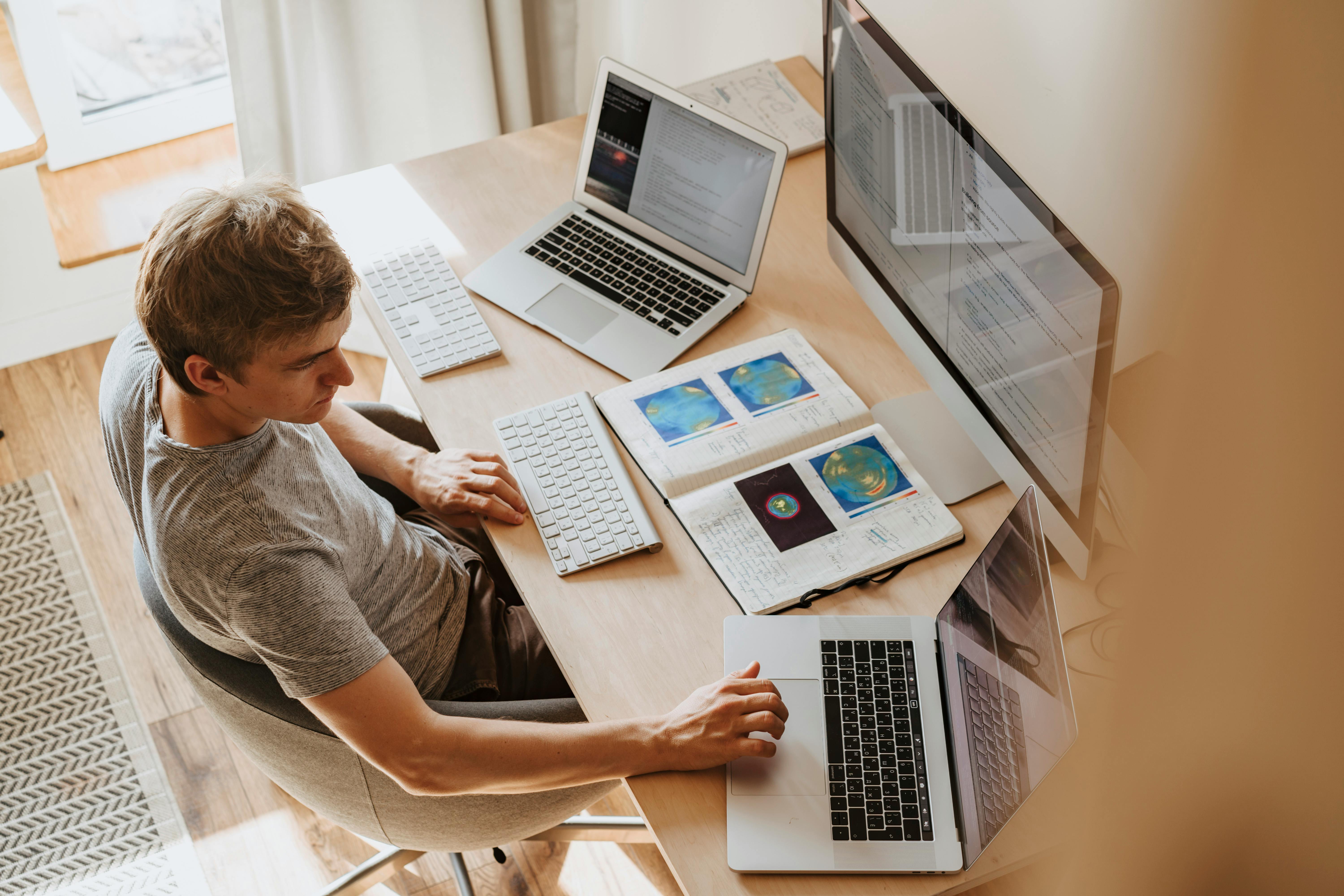
Integration with Applications
Calling Stored Procedures from Applications
Integrating stored procedures with applications involves invoking them from the application code. The method and language used to call a stored procedure may vary depending on the data access framework or programming language being used.
For example, in C# with ADO.NET, you can use the SqlCommand
class to execute a stored procedure:
using (var connection = new SqlConnection(connectionString)) { using (var command = new SqlCommand(“GetEmployeeDetails”, connection)) { command.CommandType = CommandType.StoredProcedure; command.Parameters.AddWithValue(“@EmployeeID”, 123);
connection.Open(); var reader = command.ExecuteReader(); // Process result set
} }
Passing Parameters
When calling a stored procedure from an application, you need to pass any required input parameters. The data type and structure of the parameters should match the defined parameters of the stored procedure.
Consider using parameterized queries or prepared statements provided by the data access framework to prevent SQL injection attacks. These mechanisms automatically validate and sanitize input parameters, ensuring the security and integrity of your data.
Handling Result Sets
Stored procedures can return result sets that need to be processed by the application. Result sets can consist of one or more rows and columns, depending on the specific query or logic within the stored procedure.
Applications must handle the returned data efficiently and appropriately. Different programming languages and data access frameworks provide various techniques and data structures, such as data readers, data tables, or ORMs (Object-Relational Mappers), to process and transform the result sets into usable objects or data structures within the application.
Testing and Debugging Stored Procedures
Unit Testing
Unit testing for stored procedures involves creating test cases that verify the functionality and correctness of individual procedures. Unit tests typically cover different code paths within the stored procedure, handling both normal and edge cases.
Create test scripts or use testing frameworks specifically designed for database testing to automate the execution of these unit tests. This allows you to quickly and repeatedly validate the behavior of your stored procedures, ensuring they meet the expected requirements and produce the desired output.
Integration Testing
Integration testing involves verifying the interaction and compatibility of stored procedures with other components of the system, such as data access layers, application logic, or external systems. Integration tests focus on testing the end-to-end behavior of the system, ensuring that the execution of stored procedures, along with other components, produces the expected outcome.
Consider designing test scenarios that mimic real-world scenarios and simulate different usage patterns of the stored procedures. This helps identify and resolve any integration issues, compatibility problems, or performance bottlenecks that may arise when using the stored procedures in conjunction with other application components.
Debugging Techniques
Debugging stored procedures can be challenging, as the code is executed within the database engine, not within the application code. However, most database management systems provide debugging capabilities, such as step-by-step execution, breakpoints, and variable inspection, to aid in debugging stored procedures.
Use debugging tools and IDEs specific to your database system to set breakpoints, step through the stored procedure code, and inspect the values of variables or parameters during execution. This allows you to identify and fix any logic errors, performance issues, or unexpected behavior within the stored procedures.
Migrating and Deploying Stored Procedures
Database Migration Scripts
Database migration scripts play a vital role in managing the deployment and versioning of stored procedures. Migration scripts track and apply changes to the database schema, including the creation, modification, or removal of stored procedures.
Adopting a database migration tool or framework, such as Flyway or Liquibase, simplifies the process of managing and deploying stored procedures. These tools help automate the execution of migration scripts, ensure database consistency across environments, and facilitate collaborative development by tracking and applying changes made by multiple developers.
Deployment Strategies
When deploying stored procedures to different environments, it is essential to have a well-defined deployment strategy. Use deployment automation tools or scripts to streamline the deployment process, reducing the chances of human error and promoting consistency across environments.
Consider employing techniques such as blue-green deployments or canary deployments, where new versions of stored procedures are gradually rolled out to production environments, ensuring minimal disruption to ongoing operations.
Rollback and Recovery
Deploying stored procedures introduces the possibility of errors or unforeseen issues. Therefore, it is essential to have a rollback and recovery strategy in place.
Before deploying new versions of stored procedures, make sure to take backups of the existing procedures or previous versions. This allows for a quick rollback in case of any critical issues or unintended behavior.
Monitor the deployment process closely, and have a plan in place to handle any exceptions or errors that occur during the deployment. Test the changes thoroughly in a staging environment before deploying to production, ensuring you have a stable and validated version of the stored procedures before releasing them.
Future Trends in Stored Procedures
Containerization and Orchestration
As containerization and orchestration technologies, such as Docker and Kubernetes, continue to gain popularity, there is a growing trend towards deploying and running databases and stored procedures within containerized environments.
Containerization allows for easier deployment, scaling, and portability of databases and stored procedures across different environments. Orchestration frameworks provide advanced management and scheduling capabilities, ensuring high availability and fault tolerance for stored procedure execution.
Serverless Computing
Serverless computing, also known as Function-as-a-Service (FaaS), is another trend shaping the future of stored procedures. With serverless platforms like AWS Lambda, Azure Functions, or Google Cloud Functions, you can execute stored procedures in a serverless environment, only paying for the actual execution time and resources consumed.
Serverless computing simplifies the scalability and management of stored procedures, as the infrastructure provisioning and scaling is handled by the cloud provider. This enables developers to focus solely on the logic and functionality of the stored procedures without worrying about server management or infrastructure maintenance.
Artificial Intelligence Integration
With advancements in artificial intelligence (AI) and machine learning (ML), there is an increasing opportunity to leverage these technologies within stored procedures. By integrating AI capabilities into stored procedures, you can automate or enhance various aspects of data processing, analysis, or decision-making.
Stored procedures can leverage AI algorithms for tasks such as data classification, pattern recognition, predictive analysis, or natural language processing. This allows for intelligent data processing and automation, enabling faster and more accurate insights from the database.
The future of stored procedures lies in their continued evolution and adaptation to emerging technologies, providing developers with powerful tools to enhance database functionality and efficiency. As technology progresses, it is crucial to stay updated with the latest trends and best practices to leverage the full potential of stored procedures.WordPress Post Link
Leave a Reply